How to perform Unit Testing of React Apps using JEST
Go Back to blogs page
- Date
- Author
Read Time : 4 mins
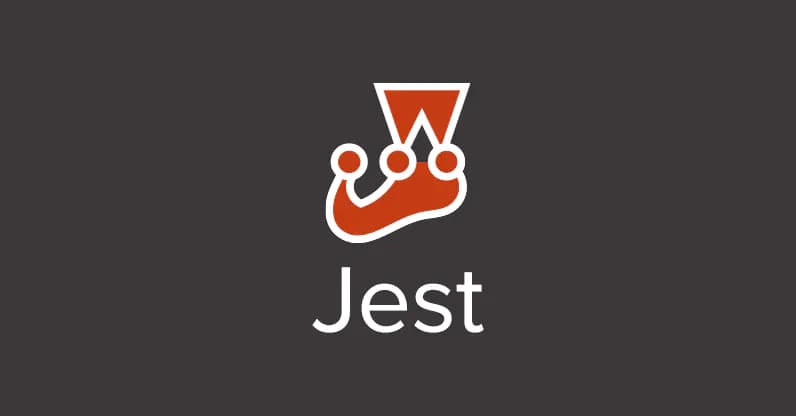
React | Jest | React Testing Library |
Introduction
Jest is a JavaScript testing framework that allows developers to run tests on JavaScript and TypeScript code and can be easily integrated with React JS
Step 1: Create a new react app
For unit testing a react app, let’s create one using the command given below:
npx create-react-app react-testing-tutorial
Open the package.json, and you will find that when you use create-react-app for creating a react project, it has default support for jest and react testing library. This means that we do not have to install them manually.
Step 2: Create a component
Let’s create a component called Counter, which simply increases and decreases a numeric value at the click of respective buttons.
import React, { useState } from "react";
const Counter = () => {
const [counter, setCounter] = useState(0);
const incrementCounter = () => {
setCounter((prevCounter) => prevCounter + 1);
};
const decrementCounter = () => {
setCounter((prevCounter) => prevCounter - 1);
};
return (
<>
<button data-testid="increment" onClick={incrementCounter}>
{" "}
+{" "}
</button>
<p data-testid="counter">{counter}</p>
<button disabled data-testid="decrement" onClick={decrementCounter}>
{" "}
-{" "}
</button>
</>
);
};
exportdefaultCounter;
Here, the important thing to note is the data-testid attributes that will be used to select these elements in the test file.
Step 3: Write a unit test for the react component
Before writing an actual unit test, let’s understand the general structure of a test block:
-
A test is usually written in a test block.
-
Inside the test block, the first thing we do is to render the component that we want to test.
-
Select the elements that we want to interact with
-
Interact with those elements
-
Assert that the results are as expected.
import { render, fireEvent, screen } from "@testing-library/react";
import Counter from "../components/Counter"; //test block
test("increments counter", () => {
// render the component on virtual dom
render(<Counter />); //select the elements you want to interact with
const counter = screen.getByTestId("counter");
const incrementBtn = screen.getByTestId("increment"); //interact with those elements
fireEvent.click(incrementBtn); //assert the expected result
expect(counter).toHaveTextContent("1");
});
Note: In order to let jest know about this test file, it’s important to use the extension .test.js.
The above test can be described as:
-
The test block can be written either using
-
The first parameter is to name the test. For example, increments counter
-
test() or it() . Either of the two methods takes two parameters:
-
The second parameter is a callback function, which describes the actual test.
-
Using the render() method from the react testing library in the above test to render the Counter component in a virtual DOM.
-
The screen property from the react testing library helps select the elements needed to test by the test ids provided earlier.
-
To interact with the button, using the fireEvent property from the react testing library in the test.
-
And finally, it is asserted that the counter element will contain a value ‘1’.
Step 4: Run the test
Run the test using the following command:
npm run test